I'd start with your enum:
enum R_P_S
{
invalid,
rock,
paper,
scissor
}
I'm not going to mention R_P_S
is a really bad name for it and that types and their members should be PascalCase
(or did I just... yeah I did), but the biggest problem here is this invalid
value. If you have 3 valid values, make the enum have, well, 3 valid values:
enum Selection
{
Rock,
Paper,
Scissors
}
You're using this invalid
value to represent the lack of a selection - when there's no selection yet...
public abstract R_P_S Choice(); //Every participant needs to have a choice. No choice about that TROLLOLOLOL
...it makes more sense to convey the lack of a value by using a nullable type - a Nullable<R_P_S>
or, as I'd put it, a Selection?
.
public abstract Selection? Choice();
That way, when the input is invalid...
if (!validEntry)
{
return R_P_S.invalid;
}
You could just return null
:
if (!validEntry)
{
return null;
}
That said, Choice()
is a bad name for a method. Try to name your methods starting with a verb: methods do something - keep nouns for types (classes).
These variables aren't needed:
R_P_S rock = R_P_S.rock;
R_P_S scissor = R_P_S.scissor;
R_P_S paper = R_P_S.paper;
And the Settings
class and its Space
member is really only used in the determineWinner
static method, to format the output. Wait. "Determine winner", "Format the output" ...how do these two things end up in the same sentence?
I bet this comment will make you think "WTF?" in a few months from now:
//1-3 2
//1-4 3
Do yourself a favor, remove the fluff:
// THE TROLL KING ENTERS
// DEEEEEEEZ NUUUTS
//Every participant needs to have a choice. No choice about that TROLLOLOLOL
Keep comments that explain why, and remove anything that says what (and especially those that say wut?!)
This comment made me wonder:
//Made it static - So it does not change on a object reference basis.
The member is static indeed, and making it static will effectively make it belong to the type, as opposed to the instance. But the type in question is a struct
- a value type. Value types don't have "object references". And value types should be immutable. In fact, Game_Info
is outright wrong. Make it a reference type (a class), and don't expose public fields - expose properties instead.
As an exercise for pushing flexibility, I'd recommend you consider how your code could be refactored to support Rock-Paper-Scissors-Lizard-Spock, or even to push it to... Rock-Paper-Scissors-Lizard-Spock-Spiderman-Batman-Wizard-Glock:
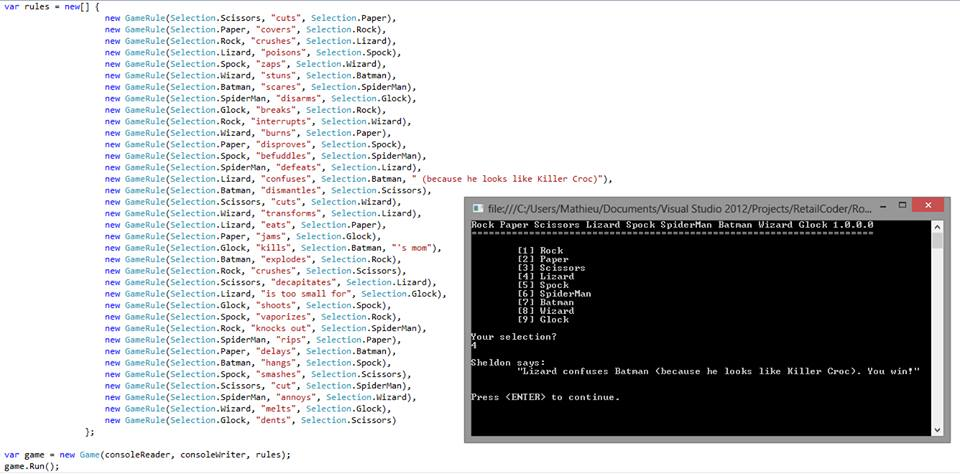